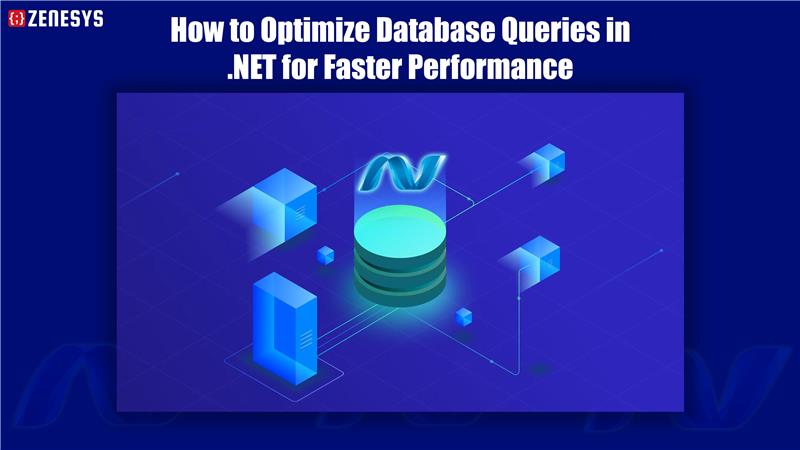
When it comes to developing applications in .NET, one of the most crucial factors influencing performance is how you manage database queries. A slow database query can result in poor user experience, and in today’s fast-paced environment, that can lead to high abandonment rates or even lost business opportunities. So, how do you optimize database queries to ensure faster performance? Let’s dive into the details.
Understanding the Core Problem
Before we begin optimizing, let’s take a moment to understand the issue. You might be working on a web application, a mobile app, or even a desktop solution, and you’ve noticed the application is slow when fetching data. This slowdown often traces back to inefficient database queries. These queries can either be poorly written, underutilized, or lack proper indexing.
I’ve observed that a lot of developers, when beginning with .NET development, often focus on getting the application to work first and then leave optimization for later. However, by focusing on proper database optimization techniques right from the start, you can avoid headaches down the line.
1. Understanding the SQL Query Plan
The first step in improving database query performance is understanding how the database engine processes your queries. A query execution plan is a blueprint of how a database engine will retrieve and manipulate data. By analyzing this plan, you can determine if your queries are efficient or if they need optimization.
For example, if you’re running a SELECT query with a JOIN clause, the database might perform a full table scan instead of an index seek, which would slow things down. You can view the execution plan in SQL Server by using the EXPLAIN statement or similar commands in other database systems.
Tip:
Using SQL Server Management Studio (SSMS), you can use the Include Actual Execution Plan option to visualize the query’s execution. This will give you insight into which operations are taking the most time.
2. Use Indexing Effectively
In many cases, poor performance is due to improper indexing. Indexes are like road signs for the database. They guide the database to find data faster. Without indexes, the database has to perform a costly full table scan to find the requested data.
However, not every column should be indexed. Think of indexing like creating shortcuts in a map—too many shortcuts can make the map cluttered and harder to read. The same goes for indexing; too many indexes slow down write operations like INSERT, UPDATE, or DELETE.
Practical Tip: Index columns that are frequently queried or used in WHERE, ORDER BY, or JOIN clauses. Also, try to use composite indexes (indexes on multiple columns) for queries that filter based on more than one column.
3. Optimize the Query Structure
Sometimes, database queries are just poorly structured, leading to inefficiencies. This is something I’ve seen in many projects where developers tend to overcomplicate queries when simpler alternatives exist.
For instance, if you’re selecting all columns (SELECT *), consider specifying only the columns you need. This simple change reduces the amount of data transferred from the database to the application, improving performance.
Example:
Instead of:
sql
CopyEdit
SELECT * FROM Employees
Use:
sql
CopyEdit
SELECT FirstName, LastName, Department FROM Employees
This cuts down on unnecessary data retrieval and speeds things up.
4. Avoid N+1 Query Problems
This is a common issue I’ve encountered when working with .NET applications that interact with relational databases. The N+1 problem occurs when your application makes multiple small database calls, resulting in many round-trips to the database. This can severely affect performance, especially in web applications.
Imagine if you’re trying to retrieve a list of authors and their books. Instead of fetching all books in one query, you might fetch the authors first, and then for each author, fetch their books in a separate query. This results in one query for authors and multiple additional queries for books.
Solution:
You can solve this by using eager loading in .NET with tools like Entity Framework. This allows you to retrieve both authors and books in a single query.
csharp
CopyEdit
var authorsWithBooks = dbContext.Authors.Include(a => a.Books).ToList();
By using Include, the related data (books) will be retrieved in the same query, preventing multiple database calls.
5. Consider Asynchronous Queries
.NET provides support for asynchronous programming through the async and await keywords. This is a game-changer when it comes to performance. Traditional, synchronous database calls can block the main thread, causing your application to become unresponsive while waiting for a database response.
By making your database queries asynchronous, you free up the application thread to do other work, improving the overall responsiveness of your application.
Here’s an example of how to use async queries in Entity Framework:
csharp
CopyEdit
public async Task<List<Employee>> GetEmployeesAsync()
{
return await dbContext.Employees.ToListAsync();
}
This ensures that the application remains responsive while the database query is being executed.
6. Batch Your Queries
If you’re executing several similar queries at once, consider batching them together. Instead of executing each query individually, you can group similar queries into one batch. This reduces the number of database round-trips and can improve performance.
In .NET, you can achieve this using bulk operations with libraries like EF Core Bulk Extensions or by manually batching operations using raw SQL.
Example:
csharp
CopyEdit
var employeesToUpdate = dbContext.Employees.Where(e => e.IsActive).ToList();
foreach (var employee in employeesToUpdate)
{
employee.Status = “Inactive”;
}
await dbContext.SaveChangesAsync();
This method batches updates into a single transaction, making it more efficient than updating each record individually.
7. Optimize Data Types
One thing I’ve learned over the years is that choosing the right data type for your database columns can have a huge impact on performance. For example, if you use a VARCHAR column for storing dates, you’re adding unnecessary overhead because it will take up more storage space and result in slower operations.
Instead, use the appropriate data types. For dates, use DATE or DATETIME types. For numbers, use the smallest numeric data type that fits your needs (e.g., INT instead of BIGINT if you don’t need such large numbers).
8. Query Caching
Caching is an essential optimization technique. With caching, you can store the results of expensive database queries in memory, reducing the number of times the same query is executed.
In .NET, you can use various caching techniques, such as MemoryCache or Distributed Cache for larger-scale applications. Here’s a simple example using MemoryCache:
csharp
CopyEdit
ObjectCache cache = MemoryCache.Default;
string cacheKey = “employeeList”;
if (cache.Contains(cacheKey))
{
return (List<Employee>)cache.Get(cacheKey);
}
var employees = dbContext.Employees.ToList();
cache.Add(cacheKey, employees, DateTimeOffset.Now.AddMinutes(10));
return employees;
By caching the results, repeated database queries are eliminated, improving performance.
9. Review Your Stored Procedures
Stored procedures can help in encapsulating SQL logic within the database, which reduces the complexity of your application code and can often be optimized more effectively by the database engine.
In .NET, you can call stored procedures through Entity Framework or ADO.NET. The benefit is that the database engine can reuse the execution plan for stored procedures, often leading to faster performance.
Example using ADO.NET:
csharp
CopyEdit
using (var command = new SqlCommand(“GetEmployees”, connection))
{
command.CommandType = CommandType.StoredProcedure;
var reader = command.ExecuteReader();
while (reader.Read())
{
// Process each record
}
}
10. Database Maintenance
I’ve come to realize that even the best-optimized queries can slow down over time if the database itself isn’t maintained. Regular maintenance tasks such as index rebuilding, updating statistics, and database cleanup can go a long way in improving database performance.
Make it a point to monitor your database’s health and perform these tasks regularly. Tools like SQL Server Management Studio (SSMS) and automated tasks within the SQL Server Agent can be set up to keep your database optimized.
Conclusion: Why You Should Hire .NET Developers for Effective Database Optimization
Optimizing database queries is no small task, and it can make or break your application’s performance. If you’re looking to achieve the best results and ensure that your application runs efficiently at scale, you may want to consider hire .NET developers who specialize in database optimization. Experienced developers can help you write efficient queries, structure your database effectively, and implement the best practices we’ve discussed.
Hiring the right professionals will allow you to focus on building features while leaving the performance optimization in expert hands. So, if you’re ready to boost your application’s performance, consider hiring .NET developers who understand the nuances of database query optimization.
By focusing on these strategies, you can ensure that your .NET application runs smoothly, scales well, and provides users with the best possible experience.